문제1) "홍길동","이길동","박길동","김길동" ,"홍길동" 데이터를 Set 인터페이스를 사용하여 저장후 원소 갯수를 출력하세요.
Set<String> set1 = new HashSet<>();
set1.add("홍길동");
set1.add("이길동");
set1.add("박길동");
set1.add("김길동");
set1.add("홍길동");
System.out.println("1번 set1의 원소 갯수:"+set1.size());
1번 set1의 원소 갯수:4 |
문제2) 정수 타입 Set을 선언 후 29, 33, 22, 33, 46, 52, 67, 78, 46 을 대입한 후
추가된 원소의 갯수와 홀수 원소의 합을 출력하세요.
Set<Integer> set2 = new HashSet<>();
set2.add(29);
set2.add(33);
set2.add(22);
set2.add(33);
set2.add(46);
set2.add(52);
set2.add(67);
set2.add(78);
set2.add(46);
int sum = 0;
for(int s : set2) {
if(s % 2 == 1) {
sum += s;
}
}
System.out.println("2번 set2의 원소 갯수:"+set2.size()+", set2의 원소 홀수의 합:"+sum);
2번 set2의 원소 갯수:7, set2의 원소 홀수의 합:129 |
문제3) 로또번호 1~45 중의 숫자중 랜덤하게 6개를 중복 없이 얻어낸 후 6개 전부 콘솔창에 출력하는 프로그래밍을 해보세요.
Set<Integer> set3 = new HashSet<>();
stop : while(true) {
int lotto = (int)(Math.random()*100)% 45 + 1;
set3.add(lotto);
if(set3.size() >= 6) {
break stop;
}
}
System.out.print("3번 set3 중복없는 로또 번호 6자리 : ");
for(int s : set3) {
System.out.print(s + " ");
}
3번 set3 중복없는 로또 번호 6자리 : 19 35 4 9 41 26 |
문제4) 상품명, 제조일, 브랜드, 가격 정보를 갖는 객체를 Set으로 관리하고자 합니다. 이때 상품명과 브랜드가 중복되는 객체는 추가 될 수 없도록 클래스의 hashCode()와 equals()를 재정의하세요.
public class Product {
private String name;
private String wDate;
private String brand;
private String price;
public Product(String name, String wDate, String brand, String price) {
this.name = name;
this.wDate = wDate;
this.brand = brand;
this.price = price;
}
@Override
public int hashCode() {
return name.hashCode() + brand.hashCode();
}
@Override
public boolean equals(Object o) {
if(o instanceof Product) {
Product p = (Product)o;
if(name.equals(p.name) && brand.equals(p.brand)) {
return true;
}
//return name.equals(p.name) && brand.equals(p.brand);
}
return false;
}
public String getName() {
return name;
}
public String getwDate() {
return wDate;
}
public String getBrand() {
return brand;
}
public String getPrice() {
return price;
}
public void setName(String name) {
this.name = name;
}
public void setwDate(String wDate) {
this.wDate = wDate;
}
public void setBrand(String brand) {
this.brand = brand;
}
public void setPrice(String price) {
this.price = price;
}
}
Set<Product> set4 = new HashSet<>();
set4.add(new Product("닭다리","2024-08-22","하림","20000"));
set4.add(new Product("닭다리","2024-08-22","마나커","18000"));
set4.add(new Product("닭다리","2024-07-10","하림","25000")); //상품명과 브랜드가 중복이기 때문에 2개만 들어감
System.out.println("4번 set4 원소의 갯수:"+set4.size());
4번 set4 원소의 갯수:2 |
문제5) 문제4에서 선언한 상품 클래스를 사용하여 Set인터페이스에 아래 데이터를 추가한 후 원소 갯수를 출력하세요.
스마트티비, 2023-10-10, LG, 4000000
스마트티비, 2023-10-10, 삼성, 4000000
스마트티비, 2023-09-24, LG, 2000000
에어컨, 2023-09-24, LG, 2000000
냉장고, 2023-08-08, 삼성, 3000000
Set<Product> set5 = new HashSet<>();
set5.add(new Product("스마트티비", "2023-10-10", "LG", "4000000"));
set5.add(new Product("스마트티비", "2023-10-10", "삼성", "4000000"));
set5.add(new Product("스마트티비", "2023-09-24", "LG", "2000000"));
set5.add(new Product("에어컨", "2023-09-24", "LG", "2000000"));
set5.add(new Product("냉장고", "2023-08-08", "삼성", "3000000"));
System.out.println("5번 set5 원소의 갯수:"+set5.size());
5번 set5 원소의 갯수:4 |
문제6) 문제5에서 선언한 Set인터페이스를 통하여 HashSet 안의 모든 데이터를 출력하세요.
System.out.println("6번:");
for(Product s : set5) {
System.out.println(s.getName() + " " + s.getwDate() + " " + s.getBrand() + " " + s.getPrice());
}
6번: 에어컨 2023-09-24 LG 2000000 냉장고 2023-08-08 삼성 3000000 스마트티비 2023-10-10 LG 4000000 스마트티비 2023-10-10 삼성 4000000 |
문제7) 사용자에게 5번의 숫자를 입력받아 입력된 수의 합을 구하세요. 이때 값은 중복될 수 없습니다.
sum = 0;
Scanner sc = new Scanner(System.in);
Set<Integer> set7 = new HashSet<>();
stop : while(true) {
System.out.print("숫자입력>");
int number = sc.nextInt();
if(set7.contains(number)) {
System.out.println("중복된 값입니다.");
continue;
}
sum += number;
set7.add(number);
if(set7.size() >= 5) {
break stop;
}
}
System.out.print("7번 set7 입력받은 5개 값 (");
for(Integer num : set7) {
System.out.print(num +" ");
}
System.out.println(")의 합:"+ sum);
sc.close();
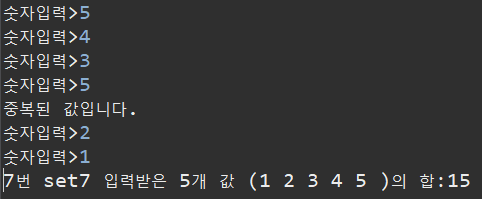
문제8) 아래 실행 예를 보고 프로그래밍 해보세요.
실행예)
-----------------------------------------------------------------------
1.데이터추가 | 2.데이터 확인 | 3. 데이터 삭제 | 4. 데이터 조회 | 5.종료
-----------------------------------------------------------------------
입력>1
문자열>apple
추가되었습니다. 현재 원소 1개
-----------------------------------------------------------------------
1.데이터추가 | 2.데이터 확인 | 3. 데이터 삭제 | 4. 데이터 조회 | 5.종료
-----------------------------------------------------------------------
입력>1
문자열>apple
중복되어 추가할수 없습니다. 현재 원소 1개
-----------------------------------------------------------------------
1.데이터추가 | 2.데이터 확인 | 3. 데이터 삭제 | 4. 데이터 조회 | 5.종료
-----------------------------------------------------------------------
입력>1
문자열>banana
추가되었습니다. 현재 원소 2개
-----------------------------------------------------------------------
1.데이터추가 | 2.데이터 확인 | 3. 데이터 삭제 | 4. 데이터 조회 | 5.종료
-----------------------------------------------------------------------
입력>1
문자열>lemon
추가되었습니다. 현재 원소 3개
-----------------------------------------------------------------------
1.데이터추가 | 2.데이터 확인 | 3. 데이터 삭제 | 4. 데이터 조회 | 5.종료
-----------------------------------------------------------------------
입력>2
문자열>apple
apple 은 존재합니다.
-----------------------------------------------------------------------
1.데이터추가 | 2.데이터 확인 | 3. 데이터 삭제 | 4. 데이터 조회 | 5.종료
-----------------------------------------------------------------------
입력>3
문자열>apple
삭제되었습니다. 남은 원소 2개
-----------------------------------------------------------------------
1.데이터추가 | 2.데이터 확인 | 3. 데이터 삭제 | 4. 데이터 조회 | 5.종료
-----------------------------------------------------------------------
입력>4
lemon, banana
입력 순서대로 출력 할 수는 없습니다.
-----------------------------------------------------------------------
1.데이터추가 | 2.데이터 확인 | 3. 데이터 삭제 | 4. 데이터 조회 | 5.종료
-----------------------------------------------------------------------
입력>5
프로그램 종료
import java.util.*;
public class Fruit {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Set<String> fruit = new HashSet<>();
stop : while(true) {
System.out.println("----------------------------------");
System.out.println("1.데이터추가 | 2.데이터 확인 | 3. 데이터 삭제 | 4. 데이터 조회 | 5.종료");
System.out.println("----------------------------------");
System.out.print("입력>");
int choice = sc.nextInt();
switch(choice) {
case 1:
System.out.print("문자열>");
String str = sc.next();
if(fruit.contains(str)) {
System.out.println("중복되어 추가할수 없습니다. 현재 원소 " + fruit.size());
break;
}
fruit.add(str);
System.out.println("추가되었습니다. 현재 원소 " + fruit.size());
break;
case 2:
System.out.print("문자열>");
str = sc.next();
if(fruit.contains(str)) {
System.out.println(str+" 은 존재합니다.");
}else {
System.out.println(str+" 은 존재하지 않습니다.");
}
break;
case 3:
System.out.print("문자열>");
str = sc.next();
if(fruit.remove(str)) {
System.out.println("삭제되었습니다. 남은 원소 " + fruit.size());
}else {
System.out.println(str+" 은 존재하지 않습니다.");
}
break;
case 4:
String fList = "";
for(String f : fruit) {
fList += f + ",";
}
fList = fList.substring(0,(fList.length()-1));
System.out.println(fList);
System.out.println("입력 순서대로 출력 할 수는 없습니다.");
break;
case 5:
System.out.println("프로그램 종료");
break stop;
default:
System.out.println("잘못 입력하셨습니다."); break;
}
}
sc.close();
}
}
문제9) 아래 실행 예를 보고 프로그래밍 해보세요. (이때, 학번, 학생명, 연락처가 동일한 학생은 추가 될 수 없습니다.)
실행예)
------------------------------------------------------------------------------------------
1.학생추가 | 2.학생 정보 확인 | 3. 학생 삭제 | 4. 학생목록 조회 | 5.학생정보 수정 | 6. 종료
------------------------------------------------------------------------------------------
입력>1
학번>001
학생명>홍길동
나이>23
주소>전주 덕진구
연락처>010-1111-1111
추가되었습니다. 현재 학생 1명
------------------------------------------------------------------------------------------
1.학생추가 | 2.학생 정보 확인 | 3. 학생 삭제 | 4. 학생목록 조회 | 5.학생정보 수정 | 6. 종료
------------------------------------------------------------------------------------------
입력>1
학번>001
학생명>홍길동
나이>26
주소>전주 완산구
연락처>010-1111-1111
이미 존재하는 학생입니다. 현재 학생 1명
------------------------------------------------------------------------------------------
1.학생추가 | 2.학생 정보 확인 | 3. 학생 삭제 | 4. 학생목록 조회 | 5.학생정보 수정 | 6. 종료
------------------------------------------------------------------------------------------
입력>1
학번>002
학생명>이길동
나이>30
주소>대전 유성구
연락처>010-1111-2222
추가되었습니다. 현재 학생 2명
------------------------------------------------------------------------------------------
1.학생추가 | 2.학생 정보 확인 | 3. 학생 삭제 | 4. 학생목록 조회 | 5.학생정보 수정 | 6. 종료
------------------------------------------------------------------------------------------
입력>1
학번>003
학생명>김길동
나이>28
주소>전주 덕진구
연락처>010-1111-3333
추가되었습니다. 현재 학생 3명
------------------------------------------------------------------------------------------
1.학생추가 | 2.학생 정보 확인 | 3. 학생 삭제 | 4. 학생목록 조회 | 5.학생정보 수정 | 6. 종료
------------------------------------------------------------------------------------------
입력>2
학번>002
학번:002
학생명:이길동
나이:30
주소:대전 유성구
연락처:010-1111-2222
------------------------------------------------------------------------------------------
1.학생추가 | 2.학생 정보 확인 | 3. 학생 삭제 | 4. 학생목록 조회 | 5.학생정보 수정 | 6. 종료
------------------------------------------------------------------------------------------
입력>3
학번>001
삭제되었습니다. 현재 학생 2명
------------------------------------------------------------------------------------------
1.학생추가 | 2.학생 정보 확인 | 3. 학생 삭제 | 4. 학생목록 조회 | 5.학생정보 수정 | 6. 종료
------------------------------------------------------------------------------------------
입력>3
학번>004
학번이 일치하는 학생이 없습니다. 현재 학생 2명
------------------------------------------------------------------------------------------
1.학생추가 | 2.학생 정보 확인 | 3. 학생 삭제 | 4. 학생목록 조회 | 5.학생정보 수정 | 6. 종료
------------------------------------------------------------------------------------------
입력>4
학생 정보
[002_이길동] 30세 대전 유성구 010-1111-2222
[003_김길동] 28세 전주 덕진구 010-1111-3333
------------------------------------------------------------------------------------------
1.학생추가 | 2.학생 정보 확인 | 3. 학생 삭제 | 4. 학생목록 조회 | 5.학생정보 수정 | 6. 종료
------------------------------------------------------------------------------------------
입력>5
학번>002
수정정보를 입력하세요.(입력하지 않은 정보는 수정되지 않습니다.
학생명>박길동
나이>32
주소>전주 덕진구
연락처>010-1111-2222
수정이 완료되었습니다.
------------------------------------------------------------------------------------------
1.학생추가 | 2.학생 정보 확인 | 3. 학생 삭제 | 4. 학생목록 조회 | 5.학생정보 수정 | 6. 종료
------------------------------------------------------------------------------------------
입력>1
학번>002
학번:002
학생명:박길동
나이:32
주소:전주 덕진구
연락처:010-1111-2222
------------------------------------------------------------------------------------------
1.학생추가 | 2.학생 정보 확인 | 3. 학생 삭제 | 4. 학생목록 조회 | 5.학생정보 수정 | 6. 종료
------------------------------------------------------------------------------------------
입력>6
프로그램 종료
import java.util.*;
public class Student {
private String no;
private String name;
private int age;
private String addr;
private String tel;
public Student(String no, String name, int age, String addr, String tel) {
this.no = no;
this.name = name;
this.age = age;
this.addr = addr;
this.tel = tel;
}
/*
이때, 학번, 학생명, 연락처가 동일한 학생은 추가 될 수 없습니다.)
*/
@Override
public int hashCode() {
return no.hashCode() + name.hashCode() + tel.hashCode();
}
@Override
public boolean equals(Object o) {
if(o instanceof Student) {
Student s = (Student)o;
if(no.equals(s.no) && name.equals(s.name) && tel.equals(s.tel)) {
return true;
}
}
return false;
}
public String getNo() { return no; }
public String getName() { return name; }
public int getAge() { return age; }
public String getAddr() {return addr;}
public String getTel() { return tel;}
public void setNo(String no) {this.no = no;}
public void setName(String name) {this.name = name;}
public void setAge(int age) {this.age = age;}
public void setAddr(String addr) {this.addr = addr;}
public void setTel(String tel) {this.tel = tel;}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Set<Student> student = new HashSet<>();
String no = "";
String name = "";
int age = 0;
String addr = "";
String tel = "";
stop : while(true) {
System.out.println("---------------------------------");
System.out.println("1.학생추가 | 2.학생 정보 확인 | 3. 학생 삭제 | 4. 학생목록 조회 | 5.학생정보 수정 | 6. 종료");
System.out.println("---------------------------------");
System.out.print("입력>");
String choice = sc.nextLine();
switch(choice) {
case "1":
System.out.print("학번>");
no = sc.nextLine();
System.out.print("학생명>");
name = sc.nextLine();
System.out.print("나이>");
age = Integer.parseInt(sc.nextLine());
System.out.print("주소>");
addr = sc.nextLine();
System.out.print("연락처>");
tel = sc.nextLine();
Student s = new Student(no,name,age,addr,tel);
if(student.contains(s)) {
System.out.println("이미 존재하는 학생입니다. 현재 학생 " + student.size() + "명");
break;
}
student.add(s);
System.out.println("추가되었습니다. 현재 학생 " + student.size() + "명");
break;
case "2":
System.out.print("학번>");
no = sc.nextLine();
boolean found = false;
for(Student s1:student) {
if(s1.getNo().equals(no)) {
System.out.println("학번:"+s1.no);
System.out.println("학생명:"+s1.name);
System.out.println("나이:"+s1.age);
System.out.println("주소:"+s1.addr);
System.out.println("연락처:"+s1.tel);
found = true;
break;
}
}
if(!found) {
System.out.println("존재하지 않는 학생입니다.");
}
break;
case "3":
System.out.print("학번>");
no = sc.nextLine();
Iterator<Student> it = student.iterator();
boolean removed = false;
while(it.hasNext()) {
Student s1 = it.next();
if (s1.getNo().equals(no)) {
//향상된 for문으로 삭제할 경우 student.remove(s1); 으로 삭제
it.remove();
System.out.println("삭제되었습니다. 현재 학생 " + student.size() + "명");
removed = true;
break;
}
}
if (!removed) {
System.out.println("존재하지 않는 학생입니다.");
}
break;
case "4":
System.out.println("학생 정보");
for(Student s1 : student) {
System.out.printf("[%s_%s] %d세 %s %s\n",s1.no,s1.name,s1.age,s1.addr,s1.tel);
}
break;
case "5":
System.out.print("학번>");
no = sc.nextLine();
boolean isS = false;
for(Student s1:student) {
if(s1.getNo().equals(no)) {
System.out.println("수정정보를 입력하세요.(입력하지 않은 정보는 수정되지 않습니다.) ");
System.out.print("학생명>");
name = sc.nextLine();
System.out.println();
System.out.print("나이>");
String ageStr = sc.nextLine();
System.out.print("주소>");
addr = sc.nextLine();
System.out.print("연락처>");
tel = sc.nextLine();
if(!name.equals("")) {
s1.setName(name);
}
if(!ageStr.equals("")) {
s1.setAge(Integer.parseInt(ageStr));
}
if(!addr.equals("")) {
s1.setAddr(addr);
}
if(!tel.equals("")) {
s1.setTel(tel);
}
isS = true;
System.out.println("수정이 완료되었습니다.");
}
}
if (!isS) {
System.out.println("존재하지 않는 학생입니다.");
}
break;
case "6":
System.out.println("프로그램 종료");
break stop;
default: System.out.println("잘못 입력하셨습니다."); break;
}
}
sc.close();
}
}
'Java' 카테고리의 다른 글
[Java] 람다식 (2) | 2024.08.27 |
---|---|
[Java] Map (0) | 2024.08.25 |
[Java] Set, Iterator (0) | 2024.08.22 |
[Java] ArrayList, List 연습 (0) | 2024.08.21 |
[Java] 컬렉션 프레임워크 (0) | 2024.08.16 |