반응형
list.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.util.*" %>
<%@ page import="frontControllerPJT.vo.*" %>
<%
List<NoticeBoardVO> boardList = (List<NoticeBoardVO>)request.getAttribute("boardList");
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Front Controller</title>
</head>
<style>
td{
text-align:center;
}
</style>
<body>
<h2>공지사항 목록 페이지</h2><hr>
<table>
<thead>
<tr>
<th>글번호</th>
<th>제목</th>
<th width="80px">작성자</th>
<th>작성일</th>
<th>조회수</th>
</tr>
</thead>
<tbody>
<!-- /board/main.do 요청시 데이터베이스 notice_board
테이블의 전체 데이터를 해당 영역에 출력하세요
-->
<%
int num = boardList.size();
for(NoticeBoardVO n : boardList){
%>
<tr>
<td width="60px;" style="color:<%= n.getTop_yn().equals("Y") ? "red" : "black" %>";>
<%
if(n.getTop_yn().equals("Y")){
%>★<%
num--;
}else{
%>
<%= num-- %>
<%
}
%>
</td>
<td>
<!--
보통 공통 관심사에 대한 가상경로들은 첫번째 uri가 같다
지금 예제는 frontcontroller 연습을 위하여
공지사항 목록과 상세에 대한 첫번째 uri를 다르게 진행합니다
-->
<a href="<%= request.getContextPath() %>/notice/view.do?nno=<%= n.getNno() %>" style="color:<%= n.getTop_yn().equals("Y") ? "red" : "black" %>";>
<%= n.getTitle() %>
</a>
</td>
<td>
<%= n.getUid() %>
</td>
<td>
<%= n.getRdate() %>
</td>
<td width="60px;">
<%= n.getHit() %>
</td>
</tr>
<%
}
%>
</tbody>
</table>
</body>
</html>
FrontController
package frontControllerPJT.controller;
/*
frontcontroller의 역할!
모든 가상경로를 제일 처음 맞이하여 역할에 맞는 컨트롤러로 기능 처리를 넘기는 중간 매개체 역할을 한다
효과 : 가상경로마다 servlet을 선언하지 않고 역할에 맞춰서 servlet을 선언하기 때문에
servlet이 무한증식하지 않고 비교적 깔끔하게 프로젝트 구조를 구성할 수 있다
해야 할 일 : 요청 uri를 분석하여 어떤 컨트롤러로 기능을 요청해야하는지 분석 후 처리
*/
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("*.do")
public class FrontController extends HttpServlet {
private static final long serialVersionUID = 1L;
public FrontController() {}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
/*
URL : 도메인을 포함한 경로
URI : 도메인을 제외한 경로
*/
System.out.println("frontcontroller 실행 url:"+request.getRequestURI());
//1. 요청 uri 정보를 가지고 온다
String uri = request.getRequestURI();
//2. 프로젝트 경로를 가지고 온다.
String contextPath = request.getContextPath();
//3. 요청 uri에서 필요없는 프로젝트 경로를 제외한 uri를 가지고 온다
String comment = uri.substring(contextPath.length()+1);
/*
/frontControllerPJT/sample/main.do에서
/frontControllerPJT/ 부분이 잘림
*/
System.out.println(comment);
//4. 3번에서 찾은 요청 경로에서 /를 기준으로 문자열을 자른다.
String[] comments = comment.split("/");
System.out.println("comments[0] : "+comments[0]);
//5. 만들어진 문자열 배열의 첫번째 문자열이 어떤 컨트롤러로 기능을 요청해야할지 결정한다.
if(comments[0].equals("sample")) {
//SampleController 에게 처리 전달
SampleController sample = new SampleController(request,response,comments);
}else if(comments[0].equals("board")) {
BoardController board = new BoardController(request,response,comments);
}else if(comments[0].equals("notice")) {
NoticeController notice = new NoticeController(request,response,comments);
}
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
NoticeController
package frontControllerPJT.controller;
import java.io.IOException;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import frontControllerPJT.util.DBConn;
import frontControllerPJT.vo.NoticeBoardVO;
public class NoticeController {
public NoticeController(HttpServletRequest request
, HttpServletResponse response
, String[] comments) throws ServletException, IOException {
if(comments[comments.length-1].equals("view.do")) {
//comments의 마지막 인덱스 값이 main.do이면 main메소드 호출
view(request,response);
}
}
public void view(HttpServletRequest request
, HttpServletResponse response) {
String nno = request.getParameter("nno");
Connection conn = null; //DB 연결
PreparedStatement psmt = null; //SQL 등록 및 실행. 보안이 더 좋음!
ResultSet rs = null; //조회 결과를 담음
PreparedStatement psmtHit = null;
//try 영역
try{
conn = DBConn.conn();
String sql = "select n.*,uid from notice_board n "
+ " inner join user u "
+ " on n.uno = u.uno "
+ " where nno=?";
psmt = conn.prepareStatement(sql);
psmt.setString(1, nno);
rs = psmt.executeQuery();
if(rs.next()){
NoticeBoardVO vo = new NoticeBoardVO();
vo.setNno(nno);
vo.setTitle(rs.getString("title"));
vo.setUid(rs.getString("uid"));
vo.setState(rs.getString("state"));
vo.setRdate(rs.getString("rdate"));
vo.setContent(rs.getString("content"));
//조회수 증가
int hit = rs.getInt("hit");
String sqlHit = "update notice_board set hit = hit + 1 where nno = ?";
hit++;
psmtHit = conn.prepareStatement(sqlHit);
psmtHit.setString(1, nno);
psmtHit.executeUpdate();
vo.setHit(hit+"");
request.setAttribute("vo", vo);
request.getRequestDispatcher("/WEB-INF/notice/view.jsp").forward(request, response);
}else {
response.sendRedirect(request.getContextPath()+"/index.jsp");
}
}catch(Exception e){
e.printStackTrace();
}finally{
try {
DBConn.close(psmtHit, null);
DBConn.close(rs, psmt, conn);
}catch(Exception e) {
e.printStackTrace();
}
}
}
}
view.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="frontControllerPJT.vo.*" %>
<%
request.setCharacterEncoding("UTF-8");
NoticeBoardVO vo = (NoticeBoardVO)request.getAttribute("vo");
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>공지사항 상세페이지</title>
</head>
<style>
button{
background-color:white;
border: 1px solid royalblue;
color: royalblue;
width:120px;
height:30px;
cursor:pointer;
}
</style>
<body>
<h2>공지사항 상세페이지</h2><hr>
<a href="<%= request.getContextPath() %>/board/main.do">
<button>글목록</button>
</a><br>
글번호 : <span><%= vo.getNno() %></span><br>
제목 : <span><%= vo.getTitle() %></span><br>
작성자 : <span><%= vo.getUid() %></span><br>
작성일 : <span><%= vo.getRdate() %></span><br>
상태 : <span><%= vo.getState() %></span><br>
조회수 : <span><%= vo.getHit() %></span><br>
내용 : <span><%= vo.getContent() %></span><br>
</body>
</html>
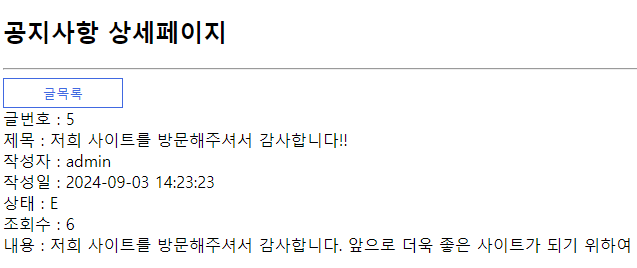
반응형
'Java' 카테고리의 다른 글
[Servlet] FrontController 회원 로그인, 내정보조회 등 (0) | 2024.10.26 |
---|---|
[Servlet] FrontController 게시글 수정하기 삭제하기 (0) | 2024.10.25 |
[Servlet] FrontController 글 목록 조회 (0) | 2024.10.20 |
[Servlet] Front Controller (0) | 2024.10.19 |
[Servlet] 게시글 삭제 (0) | 2024.10.14 |