반응형
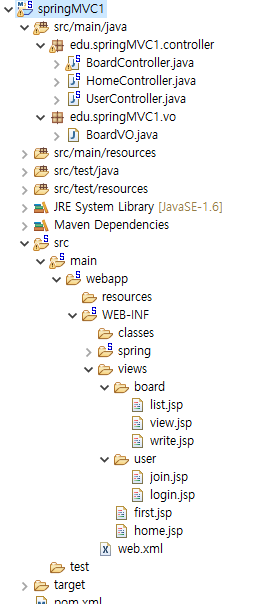
클래스 상단에 @RequestMapping을 사용하게 되면 베이스 uri를 지정할 수 있다
베이스 uri를 지정하게 되면 dispatcherservlet이 더 빨리 가상경로 매핑 메소드를 찾을 수 있다
타 가상경로 재요청할 때 "redirect: " 을 사용하는데 스프링에서는 request.getContextPath()를 생략해도 된다
return "redirect:/board/list.do";
package edu.springMVC1.controller;
import java.util.Locale;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@RequestMapping(value="/board")
@Controller
public class BoardController {
@RequestMapping(value="/list.do", method=RequestMethod.GET)
public String list() {
return "board/list";
}
@RequestMapping(value="/view.do", method=RequestMethod.GET)
public String view() {
return "board/view";
}
@RequestMapping(value="/write.do", method=RequestMethod.GET)
public String write() {
//화면 포워드
return "board/write";
}
@RequestMapping(value="/write.do", method=RequestMethod.POST)
public String writeOk() {
//타 가상경로 재요청
return "redirect:/board/list.do";
}
}
list.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>게시글 목록</title>
</head>
<body>
<a href="<%= request.getContextPath() %>">Home으로 이동</a><br>
<h2>게시글 목록</h2><hr>
<a href="view.do">게시글상세페이지로 이동</a><br>
<a href="write.do">게시글등록페이지로 이동</a>
</body>
</html>
write.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>게시글등록페이지</title>
</head>
<body>
<a href="<%= request.getContextPath() %>">Home으로 이동</a><br>
<a href="list.do">게시글 목록 페이지로 이동</a><br>
<h2>게시글등록페이지</h2><hr>
<form action="write.do" method="post">
<button>저장</button>
</form>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%@ page session="false" %>
<html>
<head>
<title>Home</title>
<meta charset="UTF-8">
</head>
<body>
<h1>
Hello world!
</h1>
<P> The time on the server is ${serverTime}. </P>
<a href="<%= request.getContextPath() %>/sample.do">sample.do로 이동</a><br>
<!-- 과제 -->
<a href="<%= request.getContextPath() %>/board/list.do">게시글 목록 페이지로 이동</a><br>
<a href="<%= request.getContextPath() %>/login.do">로그인 페이지로 이동</a><br>
<a href="<%= request.getContextPath() %>/join.do">회원가입 페이지로 이동</a><br>
</body>
</html>
값을 보낼때
Model = MVC의 M
컨트롤러에서 가공된 데이터를 모델에 전달.
model.addAttribute = request Attribute에 담는 것과 같음
package edu.springMVC1.controller;
import java.text.DateFormat;
import java.util.Date;
import java.util.Locale;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/**
* Handles requests for the application home page.
*/
@Controller
public class HomeController {
private static final Logger logger = LoggerFactory.getLogger(HomeController.class);
/**
* Simply selects the home view to render by returning its name.
*/
@RequestMapping(value = "/", method = RequestMethod.GET)
public String home(Locale locale, Model model) {
logger.info("Welcome home! The client locale is {}.", locale);
Date date = new Date();
DateFormat dateFormat = DateFormat.getDateTimeInstance(DateFormat.LONG, DateFormat.LONG, locale);
String formattedDate = dateFormat.format(date);
model.addAttribute("serverTime", formattedDate );
return "home";
}
// method = RequestMethod.GET 를 제거할경우 get이어도 post이어도 실행
@RequestMapping(value = "/sample.do", method = RequestMethod.GET)
public String sample(Model model) {
String data = "컨트롤러에서 가공한 데이터";
//키, 값
//request Attribute에 담는 것과 같음
model.addAttribute("data",data);
return "first";
}
}
first.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
String data = (String)request.getAttribute("data");
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>first jsp</title>
</head>
<body>
sample.do 포워드 되는 웹페이지 입니다<br>
<%= data %>
</body>
</html>
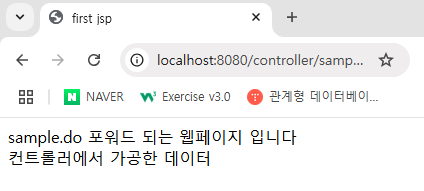
값을 받을때
보내는 쪽의 name과 일치하는 변수명으로 값을 받으면 받아짐
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>게시글등록페이지</title>
</head>
<body>
<a href="<%= request.getContextPath() %>">Home으로 이동</a><br>
<a href="list.do">게시글 목록 페이지로 이동</a><br>
<h2>게시글등록페이지</h2><hr>
<form action="write.do" method="post">
제목 : <input type="text" name="title"><br>
내용 : <textarea name="content" cols="30" rows="10"></textarea><br>
<button>저장</button>
</form>
</body>
</html>
package edu.springMVC1.controller;
import java.util.Locale;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/*
클래스 상단에 @RequestMapping을 사용하게 되면 베이스 uri를 지정할 수 있다
베이스 uri를 지정하게 되면 dispatcherservlet이 더 빨리 가상경로 매핑 메소드를 찾을 수 있다
*/
@RequestMapping(value="/board")
@Controller
public class BoardController {
@RequestMapping(value="/list.do", method=RequestMethod.GET)
public String list() {
return "board/list";
}
@RequestMapping(value="/view.do", method=RequestMethod.GET)
public String view() {
return "board/view";
}
@RequestMapping(value="/write.do", method=RequestMethod.GET)
public String write() {
//화면 포워드
return "board/write";
}
@RequestMapping(value="/write.do", method=RequestMethod.POST)
public String writeOk(String title,String content) {
/*
보내는 쪽의 name과 일치하는 변수명으로 값을 받으면 받아짐
*/
System.out.println("title: " + title);
System.out.println("content: " + content);
//타 가상경로 재요청
return "redirect:/board/list.do";
}
}
title: test content: test |
콘솔창에 한글이 깨지는 문제 발생시 기존에는 request.setCharacterEncoding을 사용하여 UTF-8로 받았지만
스프링에서는 web.xml 안에 밑의 코드를 넣어주면 된다
<!-- encoding -->
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
BoardVO
package edu.springMVC1.vo;
public class BoardVO {
private String title;
private String writer;
private String password;
private String writeDate;
private String content;
public String getTitle() { return title; }
public String getWriter() { return writer; }
public String getPassword() { return password; }
public String getWriteDate() { return writeDate;}
public String getContent() { return content; }
public void setTitle(String title) { this.title = title; }
public void setWriter(String writer) { this.writer = writer; }
public void setPassword(String password) { this.password = password; }
public void setWriteDate(String writeDate) { this.writeDate = writeDate; }
public void setContent(String content) { this.content = content; }
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>게시글등록페이지</title>
</head>
<body>
<a href="<%= request.getContextPath() %>">Home으로 이동</a><br>
<a href="list.do">게시글 목록 페이지로 이동</a><br>
<h2>게시글등록페이지</h2><hr>
<form action="write.do" method="post">
제목 : <input type="text" name="title"><br>
작성자 : <input type="text" name="writer"><br>
비밀번호 : <input type="password" name="password"><br>
작성일 : <input type="date" name="writeDate"><br>
내용 : <textarea name="content" cols="30" rows="10"></textarea><br>
<button>저장</button>
</form>
</body>
</html>
BoardController
컨트롤러에서 매개변수의 명칭과 파라미터의 키값이 같으면 자동 매핑되어 값이 대입된다
이때 매개변수를 vo로 둘 경우 vo의 필드명과 파라미터의 키값이 같으면 자동 매핑되어 객체를 생성한다
@RequestMapping(value="/write.do", method=RequestMethod.POST)
public String writeOk(BoardVO boardVO) {
System.out.println(boardVO.getTitle());
System.out.println(boardVO.getWriter());
System.out.println(boardVO.getPassword());
System.out.println(boardVO.getWriteDate());
System.out.println(boardVO.getContent());
//타 가상경로 재요청
return "redirect:/board/list.do";
}
제목~~ 작성자1 1234 2024-11-19 내용~~ |
BoardVO에 넣기는 애매한 값이 있을 경우 별도로 받을수있다
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>게시글등록페이지</title>
</head>
<body>
<a href="<%= request.getContextPath() %>">Home으로 이동</a><br>
<a href="list.do">게시글 목록 페이지로 이동</a><br>
<h2>게시글등록페이지</h2><hr>
<form action="write.do" method="post">
제목 : <input type="text" name="title"><br>
작성자 : <input type="text" name="writer"><br>
비밀번호 : <input type="password" name="password"><br>
<!--작성일 : <input type="date" name="writeDate"><br> -->
아무거나 : <input type="text" name="other"><br>
내용 : <textarea name="content" cols="30" rows="10"></textarea><br>
<button>저장</button>
</form>
</body>
</html>
BoardVO를 받는 곳 뒤에 String 으로 그 값을 추가로 받으면 된다
@RequestMapping(value="/write.do", method=RequestMethod.POST)
public String writeOk(BoardVO boardVO,String other) {
System.out.println(boardVO.getTitle());
System.out.println(boardVO.getWriter());
System.out.println(boardVO.getPassword());
/* System.out.println(boardVO.getWriteDate()); */
System.out.println(boardVO.getContent());
System.out.println(other);
//타 가상경로 재요청
return "redirect:/board/list.do";
}
반응형
'Spring' 카테고리의 다른 글
[Spring] MVC 연습 (0) | 2024.11.28 |
---|---|
[Spring] Spring MVC (2) | 2024.11.27 |
[Spring] 스프링 MVC 프로젝트 (0) | 2024.11.24 |
[Spring] AOP 어노테이션 (0) | 2024.11.23 |
[Spring] joinpoint (0) | 2024.11.22 |