반응형
XML
- 다목적 마크업 언어
- HTML 형식처럼 태그로 데이터를 표현
- 데이터를 저장하고 전달할 목적으로만 만들어졌다
- HTML 태그처럼 미리 정의되어 있지 않고, 사용자가 직접 정의할 수 있다
- 서로 다른 시스템끼지 다양한 종류의 데이터를 손쉽게 교활할 수 있도록 해준다
- 텍스트 형식의 언어로 모든 XML 문서는 유니코드 문자로만 이루어진다
- 새로운 태그를 만들어 추가해도 동작하므로, 확장성이 좋다
Servers 폴더의 web.xml 파일의 기본 설정이 index.??? 으로 되어있기 때문에 프로젝트 경로로 이동시 index 파일의 주소를 대문페이지로 들어가게 된다


<?xml version="1.0" encoding="UTF-8"?>
<books>
<book>
<name>이것이 자바다</name>
<publisher>한빛미디어</publisher>
<author>홍길동</author>
<price>34,000원</price>
</book>
<book>
<name>이것이 MySQL이다</name>
<publisher>한빛미디어</publisher>
<author>김길동</author>
<price>30,000원</price>
</book>
<book>
<name>명품 웹 프로그래밍</name>
<publisher>명품미디어</publisher>
<author>박길동</author>
<price>32,000원</price>
</book>
</books>


도서 정보 테이블로 출력하기
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>xml과 json 데이터 ajax로 다루기</title>
<script src="js/jquery-3.7.1.js"></script>
<script>
function callBooksXML(){
$.ajax({
url : "data/books.xml",
type : "get",
success : function(xml){
//console.log(xml);
let xml_ = $(xml); //document 객체를 jQuery객체로 변환
console.log(xml_);
let books = xml_.find("books").children("book");
console.log(books);
let html = "";
html += "<table border='1'>";
html += "<tr>";
html += " <th>제목</th>";
html += " <th>출판사</th>";
html += " <th>저자</th>";
html += " <th>가격</th>";
html += "</tr>";
books.each(function(){
let name = $(this).children("name").text();
let publisher = $(this).children("publisher").text();
let author = $(this).children("author").text();
let price = $(this).children("price").text();
console.log(name+" : "+publisher+" : "+author+" : "+price);
html += "<tr>";
html += " <td>"+ name + "</td>";
html += " <td>"+ publisher + "</td>";
html += " <td>"+ author + "</td>";
html += " <td>"+ price + "</td>";
html += "</tr>";
});
html += "</table>";
$("#booksList").html(html);
}
});
}
</script>
</head>
<body>
<h1>xml과 json 데이터 ajax로 다루기</h1>
<button onclick="callBooksXML();">books xml 요청</button>
<div id="booksList"></div>
</body>
</html>
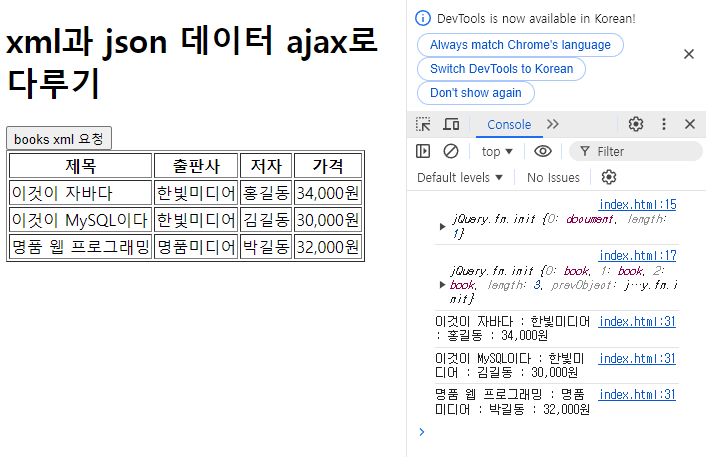
커피 메뉴 테이블로 출력하기
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>xml과 json 데이터 ajax로 다루기</title>
<script src="js/jquery-3.7.1.js"></script>
<script>
/* menu xml */
function callMenuXML(){
$.ajax({
url : "data/menu.xml",
type : "get",
success : function(xml){
let xml_ = $(xml);
let menus = xml_.find("menu").children("name").text();
console.log(menus);
let html = "";
html += "<table border='1'>";
html += "<caption>" + menus + "</caption>";
html += "<tr>";
html += " <th>이름</th>";
html += " <th>가격</th>";
html += "</tr>";
let menu = xml_.find("menu").children("submenu");
menu.each(function(){
let name = $(this).children("name").text();
let price = $(this).children("price").text();
html += "<tr>";
html += " <td>"+ name + "</td>";
html += " <td>"+ price + "</td>";
html += "</tr>";
});
html += "</table>";
$("#menuList").html(html);
}
});
}
</script>
</head>
<body>
<h1>xml과 json 데이터 ajax로 다루기</h1>
<button onclick="callMenuXML();">menu xml 요청</button>
<div id="menuList"></div>
</body>
</html>

JSON
- 자바스크립트에서 사용하는 객체 형태로 데이터를 표현하는 방법이다.
- 데이터를 저장하거나 전송할 때 많이 사용되는 경량의 DATA 교환 형식이다.
- 데이터 포맷일 뿐이며 어떠한 통신 방법도, 프로그래밍 문법도 아닌 단순히 데이터를 표시하는 표현 방법일 뿐이다
-
자바스크립트 객체 표기법과 아주 유사하다.
- 자바스크립트를 이용하여 JSON 형식의 문서를 쉽게 자바스크립트 객체로 변환할 수 있는 이점이 있다
- .json 으로 요청 경로는 jQuery에서 자동으로 자바스크립트 객체로 변환하여 데이터를 반환
이클립스에서 JSON 파일 만들기

만들어지면 Untitiled 1이라는 이름으로 파일이 열리는데 스페이스 눌러서 한칸 띄고 ctrl+s 로 저장하려 할 경우
아래와 같은 창이 뜬다. 만들고자하는 폴더 선택해주고 이름을 지정해주면 된다


books.json을 테이블로 출력하기
books .json
[
{
"name" : "이것이 자바다" ,
"publisher" : "한빛미디어",
"author" : "홍길동",
"price" : "34,000원"
}
,{
"name" : "이것이 MySQL이다" ,
"publisher" : "한빛미디어",
"author" : "김길동",
"price" : "30,000원"
}
,{
"name" : "명품 웹 프로그래밍" ,
"publisher" : "명품미디어",
"author" : "박길동",
"price" : "32,000원"
}
]
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>xml과 json 데이터 ajax로 다루기</title>
<script src="js/jquery-3.7.1.js"></script>
<script>
/* json : books */
function callBooksJSON(){
$.ajax({
url : "data/books.json",
type : "get",
success : function(json){
//.json 으로 요청 경로는 jQuery에서 자동으로 자바스크립트 객체로 변환하여 데이터를 반환
//let json_ = JSON.parse(json);
console.log(json);
let html = "";
html += "<table border='1'>";
html += "<tr>";
html += " <th>제목</th>";
html += " <th>출판사</th>";
html += " <th>저자</th>";
html += " <th>가격</th>";
html += "</tr>";
for(let item of json){
console.log(item.name);
console.log(item.publisher);
console.log(item.author);
console.log(item.price);
console.log("-------------")
html += "<tr>";
html += " <td>"+ item.name + "</td>";
html += " <td>"+ item.publisher + "</td>";
html += " <td>"+ item.author + "</td>";
html += " <td>"+ item.price + "</td>";
html += "</tr>";
}
html += "</table>";
$("#booksListJSON").html(html);
}
});
}
</script>
</head>
<body>
<h1>xml과 json 데이터 ajax로 다루기</h1>
<button onclick="callBooksJSON();">books json 요청</button>
<div id="booksListJSON"></div>
</body>
</html>

menu.json을 테이블로 출력하기
menu.json
[{
"name" : "커피류",
"sub" : [{
"name" : "아메리카노",
"price" : "2,000원"
},{
"name" : "카페라떼",
"price" : "3,000원"
},{
"name" : "카푸치노",
"price" : "3,500원"
}]
}]
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>xml과 json 데이터 ajax로 다루기</title>
<script src="js/jquery-3.7.1.js"></script>
<script>
/* json : menu */
function callMenuJSON(){
$.ajax({
url : "data/menu.json",
type : "get",
success : function(json){
console.log(json[0].name);
let html = "";
html += "<table border='1'>";
html += "<caption>" + json[0].name + "</caption>";
html += "<tr>";
html += " <th>이름</th>";
html += " <th>가격</th>";
html += "</tr>";
let sub = json[0].sub;
console.log(sub);
for(let item of sub){
html += "<tr>";
html += " <td>"+ item.name + "</td>";
html += " <td>"+ item.price + "</td>";
html += "</tr>";
}
html += "</table>";
$("#menuListJSON").html(html);
}
});
}
</script>
</head>
<body>
<h1>xml과 json 데이터 ajax로 다루기</h1>
<button onclick="callMenuJSON();">menu json 요청</button>
<div id="menuListJSON"></div>
</body>
</html>

반응형
'JavaScript, jQuery, Ajax' 카테고리의 다른 글
[JS] 다크모드, 라이트모드 (0) | 2024.10.27 |
---|---|
[Ajax] Ajax를 활용하여 요청페이지로 파라미터 보내기 (0) | 2024.09.11 |
[Ajax] jQuery를 사용하여 Ajax 사용 (0) | 2024.09.09 |
[Ajax] 자바스크립트를 사용하여 Ajax 사용 (2) | 2024.09.08 |
[JQUERY] 다운받기 (0) | 2024.08.28 |